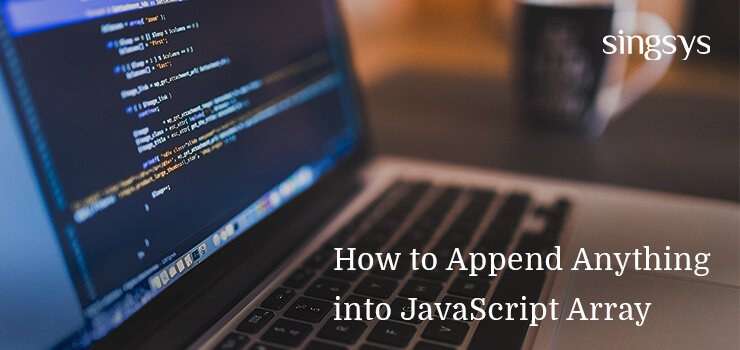
The push() method is used for adding one or more elements at the end of an array and returns the new length of the array. In order to add items at beginning of the array unshift() method is used. It can be used with call() or apply() with objects that are similar to arrays. The push method applies the length property to identify pint of inserting the given values. When the length property is not converted into a number 0 is used as index. This considers the possibility of length being nonexistent hence in such scenario length will be created.
Syntax:
arr.push([element1[, …[, elementN]]])
Code to Append Anything into JavaScript Array
// initialize array
var arr = [
“Hi”,
“Hello”,
“Bonjour”
];
// appending new value to the array
arr.push(“Hola”);
Now array is
var arr = [
“Hi”,
“Hello”,
“Bonjour”,
“Hola”
];
// appending multiple values to the array
arr.push(“Salut”, “Hey”);
Now array is
var arr = [
“Hi”,
“Hello”,
“Bonjour”,
“Hola”,
“Salut”,
“Hey”
];
// display all the values
for (var i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
Output:
Hi
Hello
Bonjour
Hola
Salut
Hey
In order to add items from one array to another array you may use Array.concat():
var arr = [
“apple”,
“banana”,
“cherry”
];
arr = arr.concat([
“dragonfruit”,
“elderberry”,
“fig”
]);
console.log(arr);
Output:
[“apple”, “banana”, “cherry”, “dragonfruit”, “elderberry”, “fig”]
Looking forward to respond to your queries and comments regarding appending anything in JavaScript.
About Singsys Pte. Ltd. Singsys is a solution provider that offer user friendly solution on cutting edge technologies to engage customers and boost your brand online results from a set of certified developers, designers who prefer optimized utilization of the available resources to align client’s idea with their skillset to reflect it into a Mobile application, Web application or an E-commerce solution
You may be interested in following:
- Random Whole Number Generation in Javascript Within a Range
- Step by Step Splitting of String in Java
- 5 Best JavaScript Testing Tools for Web Developers
- Advantages of Object Oriented JavaScript
Related Posts...
Javascript
Nov 23rd, 2023
In today’s digital landscape, a Graphical User Interface (GUI) is a pivotal element in software applications. It’s the intermediary platform where users interact with the software through visual elements like […]
Read more
Jun 20th, 2023
AngularJS, a popular JavaScript framework developed by Google, has gained significant recognition in the web development community. With its powerful features and robust architecture, AngularJS offers numerous compelling reasons to […]
Read more
Jun 1st, 2023
Exciting news for developers and enthusiasts in the JavaScript ecosystem! Node.js, the popular open-source runtime environment, has recently released its highly anticipated version 20. Packed with new features, enhancements, and […]
Read more