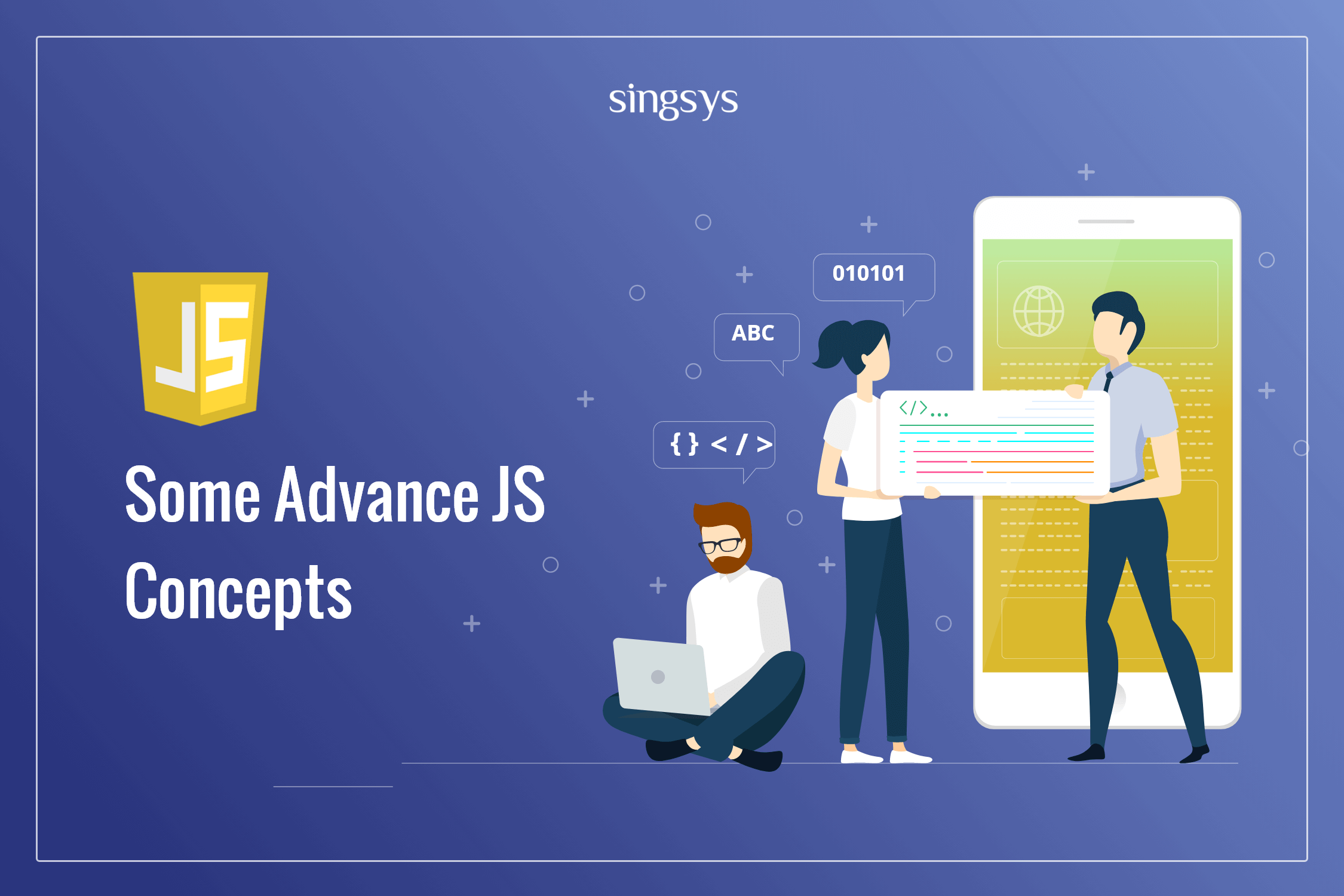
This article is created to help new developers and intermediate developers understand more about some advanced javascript concepts, so that they can create more optimized and reliable functionalities.
Use of “Closures” for extending variable scope
A closure is the combination of a function bundled together (enclosed) with references to its surrounding state. In other words, a closure gives you access to an outer function’s scope from an inner function. In JavaScript, closures are created every time a function is created, at function creation time.
To create a closure, you nest a function inside of a function. That inner function has access to all variables in its parent function’s scope. This comes in handy when creating methods and properties in object oriented scripts.
The below example demonstrates how to use a closure:
function outerFunction() {
this.variable1 = “value1”;
this.variable2 = “value2”;
var newValue = this.variable1;
this.innerFunction = function() {
innerFunctionValue = newValue;
return innerFunctionValue;
};
}
var objectInstance = new outerFunction();
console.log(objectInstance.innerFunction());
All functions have access to the global scope, all functions have access to the scope above them, these types of functions are called “Nested Functions”. The innerFunction() has access to the newValue variable in the outerFunction(). The function called in console.log is a nested function and it can access the value in the variable newValue even though the variable is not within the scope of the function or method. A closure is created anytime a function is nested inside another function and uses the variables from the parent scope.
Callback functions
A callback is a function passed as an argument to another function, this technique allows a function to call another function and can run after another function has finished. JavaScript functions are executed in the sequence they are called not in the sequence they are defined in. Sometimes you would like to have a better understanding and control over when to execute a function.
For example creating a function without a callback function :
function Output(value) {
console.log(value);
}
function sum(value1, value2) {
var result = value1 + value2;
return result;
}
var res = sum(9, 6);
Output(res);
And if you change the program and call output inside the sum() function.
function Output(value) {
console.log(value);
}
function sum(value1, value2) {
var result = value1 + value2;
Output(result);
}
sum(9, 6);
The problem with the first example is that you have to call two functions to display the result. The problem with the second example, is that you cannot prevent the sum() function from displaying the result.
Using a callback, you could call the sum function with a callback, and let the sum function run the callback after the calculation is finished:
function Output(value) {
console.log(value);
}
function sum(value1, value2, Callback) {
var result = value1 + value2;
Callback(result);
}
sum(9, 6, Output);
In the above example Output() is a callback function that is passed in a sum() function. A callback function is used where one function has to wait for another function.
Invoking functions
Invoking a function as a global function, causes the value of this to be the global object. Using the window object as a variable can easily crash your program. In Js you can also define functions as object methods.
As Method
The below example shows how we can define a function as an object method
Const newObject = {
company: “Abc”,
location: “myLocation”,
established: “2014”,
description: function() {
return this.company + “ is located in ” + this.company + ” and it was established in ” + this.established +”.”;
}
}
newObject.description();
In the above example newObject is an object with three properties: company, location and established, and a method description.
The description method is a function that belongs to the object newObject who is the owner of the function. The object that calls it is the object that “owns” the JavaScript code. In this case the value of this is newObject.
As Function Constructor
If a function invocation is preceded with the new keyword, it is a constructor invocation that looks like you create a new function, but since JavaScript functions are objects, you actually create a new object.
function student(arg1, arg2) {
this.name= arg1;
this.class = arg2;
}
const newObject = new student(“Linda Gray”, “Play school”);
newObject.name;
A constructor invocation creates a new object. The new object inherits the properties and methods from its constructor.
In the above case student is the constructor and newObject is the object created for calling the constructor, now when you call newObject.name it will return “Linda Gray”.
Conclusion
I hope these concepts provide beginning and intermediate coders with an overview of a few fairly advanced JavaScript tactics that they can implement in future projects or experiments.
Related Posts...
JavascriptjQueryTechnologiesWeb DesignWebsite developmentWhat is New!What's Hot
Apr 18th, 2024
Your corporate website is a critical component in shaping perception of your brand. Discover how elements like design, content, and UX influence how customers view your company. We break down key strategies to align your site with your brand identity.
Read more
Apr 11th, 2024
Discover how Singsys’ expertise in website development can help your business succeed with responsive and intuitive websites. Enhance user experience and boost conversions today!
Read more
Apr 2nd, 2024
As consumers’ expectations continue to rise, delivering an exceptional user experience (UX) has become a key differentiator for businesses seeking to gain a competitive edge.
Read more